Trending documentation
IBM watsonx
Build, govern, and manage your data for generative AI solutions.
IBM Cloud Pak for Business Automation
Use pre-integrated automation technologies to design, build, and run automation applications and services on the cloud.
IBM Cloud Pak for Data
Collect, organize, analyze, and operationalize data and AI throughout your business within a single, flexible interface.
IBM Cloud Pak for Integration
Use pre-integrated automation technologies to design, build, and run automation applications and services on the cloud.
IBM Cloud Pak for AIOps
Proactively avoid incidents and accelerate remediation using advanced, explainable AI across the ITOps toolchain.
View all products in IBM Documentation
IBM Cloud Paks
IBM Cloud® Paks are AI-powered software for hybrid clouds that can help you fully implement intelligent workflows in your business to accelerate digital transformation.
Explore the docsFeatured documentation
IBM Documentation for offline environments
Do you work in a Dark shop or Airgap environment where you don't have access to the internet, but still need to use documentation to troubleshoot problems or reference guides?
We have built an offline application to be able to view your product documentation in environments without internet access.
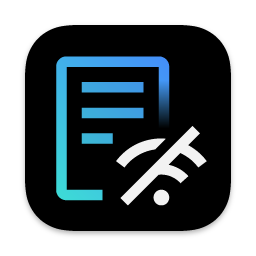
Resources
IBM Support Portal
Get assistance for the IBM products, services and software you own.
IBM Fix Central
Provides fixes and updates for your system's software, hardware, and operating system.
IBM Passport Advantage
Provides you with secure access to software downloads and media.
IBM Redbooks
Find best practices for integrating IBM technologies.
IBM Developer
Explore technical topics, find trial software and join the community.
IBM Training
Explore, learn and succeed with IBM training and certification.